It's a exercise to demonstrate the effects of various
PorterDuff.Mode. You can try it interactively.
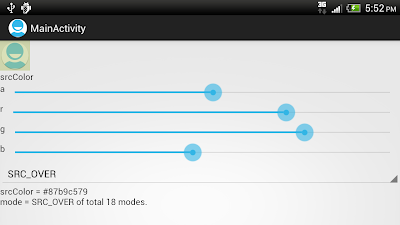
package com.example.androidcolorfilter;
import android.os.Bundle;
import android.app.Activity;
import android.graphics.Color;
import android.graphics.PorterDuff;
import android.graphics.PorterDuffColorFilter;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.SeekBar;
import android.widget.SeekBar.OnSeekBarChangeListener;
import android.widget.Spinner;
import android.widget.TextView;
public class MainActivity extends Activity {
ImageView imageView;
SeekBar alphaBar, redBar, greenBar, blueBar;
Spinner modeSpinner;
TextView colorInfo;
PorterDuff.Mode[] optMode = PorterDuff.Mode.values();
String[] optModeName;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = (ImageView)findViewById(R.id.iv);
alphaBar = (SeekBar)findViewById(R.id.bar_a);
redBar = (SeekBar)findViewById(R.id.bar_r);
greenBar = (SeekBar)findViewById(R.id.bar_g);
blueBar = (SeekBar)findViewById(R.id.bar_b);
colorInfo = (TextView)findViewById(R.id.colorinfo);
alphaBar.setOnSeekBarChangeListener(colorBarChangeListener);
redBar.setOnSeekBarChangeListener(colorBarChangeListener);
greenBar.setOnSeekBarChangeListener(colorBarChangeListener);
blueBar.setOnSeekBarChangeListener(colorBarChangeListener);
modeSpinner = (Spinner)findViewById(R.id.mode);
prepareOptModeName();
ArrayAdapter<String> modeArrayAdapter = new ArrayAdapter<String>(
this,
android.R.layout.simple_spinner_item,
optModeName);
modeArrayAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item );
modeSpinner.setAdapter(modeArrayAdapter);
modeSpinner.setSelection(0);
modeSpinner.setOnItemSelectedListener(onModeItemSelectedListener);
setPorterDuffColorFilter(imageView);
}
private void prepareOptModeName(){
optModeName = new String[optMode.length];
for(int i = 0; i < optMode.length; i++){
optModeName[i] = optMode[i].toString();
}
}
OnSeekBarChangeListener colorBarChangeListener
= new OnSeekBarChangeListener(){
@Override
public void onProgressChanged(SeekBar arg0, int arg1, boolean arg2) {
setPorterDuffColorFilter(imageView);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
};
OnItemSelectedListener onModeItemSelectedListener
= new OnItemSelectedListener(){
@Override
public void onItemSelected(AdapterView<?> arg0, View arg1, int arg2,
long arg3) {
setPorterDuffColorFilter(imageView);
}
@Override
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
}
};
private void setPorterDuffColorFilter(ImageView iv){
int srcColor = Color.argb(
alphaBar.getProgress(),
redBar.getProgress(),
greenBar.getProgress(),
blueBar.getProgress());
PorterDuff.Mode mode = optMode[modeSpinner.getSelectedItemPosition()];
PorterDuffColorFilter porterDuffColorFilter = new PorterDuffColorFilter(srcColor, mode);
iv.setColorFilter(porterDuffColorFilter);
colorInfo.setText(
"srcColor = #" + Integer.toHexString(srcColor) +"\n" +
"mode = " + String.valueOf(mode.toString()) + " of total " + String.valueOf(optMode.length) + " modes.");
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<ImageView
android:id="@+id/iv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="srcColor"
android:layout_alignParentLeft="true"/>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_a"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="a"
android:layout_alignParentLeft="true"/>
<SeekBar
android:id="@+id/bar_a"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="255"
android:layout_toRightOf="@id/text_a"
android:layout_alignParentRight="true"/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_r"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="r"
android:layout_alignParentLeft="true"/>
<SeekBar
android:id="@+id/bar_r"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="255"
android:layout_toRightOf="@id/text_r"
android:layout_alignParentRight="true"/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_g"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="g"
android:layout_alignParentLeft="true"/>
<SeekBar
android:id="@+id/bar_g"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="255"
android:layout_toRightOf="@id/text_g"
android:layout_alignParentRight="true"/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_b"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="b"
android:layout_alignParentLeft="true"/>
<SeekBar
android:id="@+id/bar_b"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="255"
android:progress="255"
android:layout_toRightOf="@id/text_b"
android:layout_alignParentRight="true"/>
</RelativeLayout>
<Spinner
android:id="@+id/mode"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/colorinfo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
Download the files.
0 comments:
Post a Comment